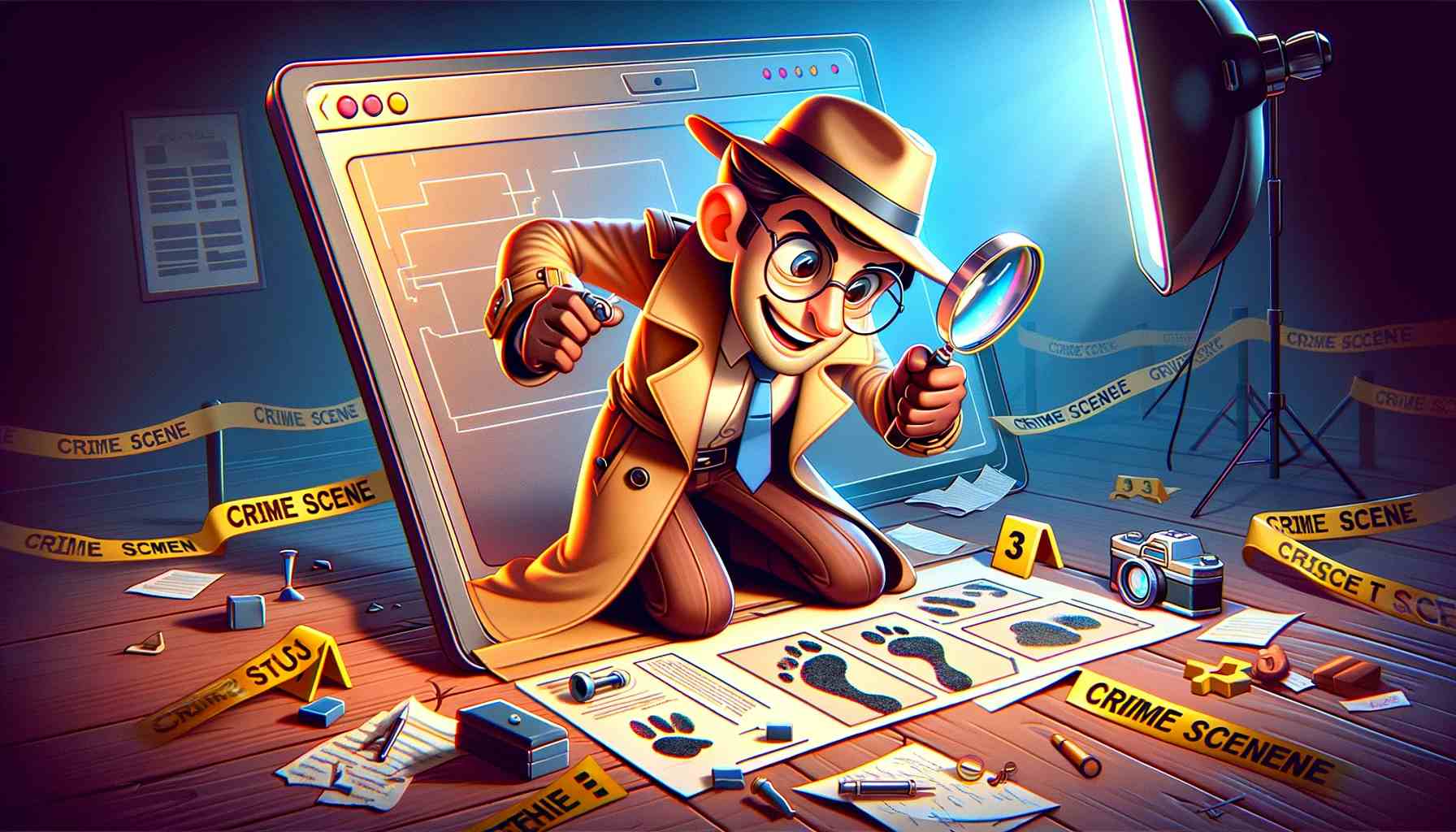
In JavaScript, working with objects is a fundamental aspect of developing dynamic web applications. A common task you might encounter is checking whether a specific key exists within an object. This capability is crucial for validating data, avoiding errors in your code, and ensuring that your application logic runs as expected. This article explores several methods to perform this check efficiently, catering to both beginners and seasoned JavaScript developers.
Using the in
Operator
The in
operator is a straightforward way to check if a key exists in an object. It returns true
if the specified property is in the object, and false
otherwise.
const person = {
name: "John Doe",
age: 30
};
console.log('name' in person); // true
console.log('address' in person); // false
This method is particularly useful for its simplicity and readability, making your code easy to understand at a glance.
The hasOwnProperty
Method
Every JavaScript object comes with the hasOwnProperty
method. This method checks if an object has a specific property as its own property (as opposed to inheriting it). It's a safe way to check for the existence of a property without accidentally checking the object's prototype chain.
const person = {
name: "John Doe",
age: 30
};
console.log(person.hasOwnProperty('name')); // true
console.log(person.hasOwnProperty('toString')); // false
While toString
exists as a method because it's inherited from Object.prototype
, hasOwnProperty
correctly identifies that it's not a direct property of the person
object.
Using the Object.keys()
Method
Another approach involves using the Object.keys()
method, which returns an array of an object's own property names. You can then check if the key exists by seeing if it's included in the array.
const person = {
name: "John Doe",
age: 30
};
const keys = Object.keys(person);
console.log(keys.includes('name')); // true
console.log(keys.includes('address')); // false
This method is particularly useful when you need to work with the keys of an object beyond just checking for the existence of a specific key, offering flexibility for more complex scenarios.
Optional Chaining (ES2020+)
With the introduction of optional chaining in ES2020, you can now check for the existence of a key more succinctly, especially if accessing nested properties.
const person = {
name: "John Doe",
contact: {
email: "johndoe@example.com"
}
};
console.log(person.contact?.email ? true : false); // true
console.log(person.contact?.phone ? true : false); // false
This method not only checks for the existence of a key but also prevents runtime errors if the parent object or property is undefined
or null
.
Conclusion
Checking if a key exists in a JavaScript object is a common task with multiple solutions, each suited to different scenarios. Whether you prefer the simplicity of the in
operator, the precision of hasOwnProperty
, the utility of Object.keys()
, or the modern approach of optional chaining, JavaScript offers the flexibility to handle this check in ways that best fit your coding style and requirements. Understanding these methods enhances your ability to manipulate and validate object data, a crucial skill in web development.